Beginner’s approach to understand & build the Cloud APIs — Build step by step with REST API using Golang — Part 1
In this article, i am explaining a very simple way to understand REST API which is used in building cloud system and other services using golang . I assume that you have a very basic knowledge of programming and open source operating system like Linux.
API is the acronym for Application Programming Interface, which is a software intermediary that allows two applications to talk to each other
A REST API (also known as RESTful API) is an application programming interface (API or web API) that conforms to the constraints of REST architectural style and allows for interaction with RESTful web services. REST stands for representational state transfer.
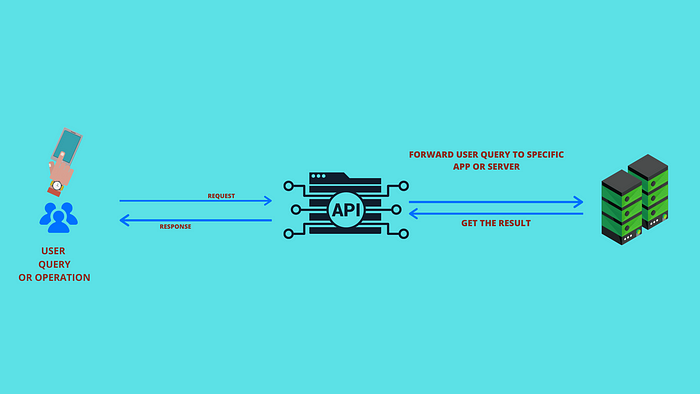
We are assuming IaaS ( Infrastructure as a Service) cloud in this example, where user can also demand hardware resources.
In a nutshell, i am covering below points in this story:
- Creating a golang service on a linux node.
- Making this available to user via REST API Calls via Postman, CURL or Browser.
- Routing user’s api via mux router module in golang.
- Getting the required resources from the infrastructure and various hosts and VMs on user’s request.
- Sending back the response to user.
Pre-requisite for the hands-on:
- Linux/Mac system
- Linux host
- Postman tool
- Curl command line tool
- Web browser
- Password less ssh connection to host (optional)
- Golang execution environment setup
First we need to choose or create an API server which will handle user request to handle cloud resources like VM , Storage, Database etc.
I am using a flask server which handles API request from user.
Below is the code snippet which will handle API request to create VM.
r.HandleFunc(“/createvm/template/{templatename}”, CreateVM).Methods(“POST”)
We will use golang for serving the API requests via CRUD ( CREATE , READ, UPDATE , DELETE ) from users or customers.
Below Fig shows how a API server serves the User’s request via REST call and then further forward it to specific hosts for execution and getting the desired results.

Below is the code snippet of golang serving the API requests and providing information to user about the type of resource requested.
In Below example ipaddress of host is requested from user via GET.
Create a file called rest_api.go as below:
Execute this using below command:
$go run rest_api.go


Fig 3. Shows User queries about VM availability and storage and networking details and API server serves this query via command executed via ssh on VM and then response is returned to the user.
Identifying the user request and then executing the query.
In Fig 1 and 3 we have only considered a query from user but what will happen if user want to create or delete some resources on VM i.e creating or deleting a volume, files, folders, nic card etc .
Then, we have to identify it via POST,PUT,DELETE REST APIs
In Fig 4, we have shown how we can use mux to identify which type of request is coming from user via front end.
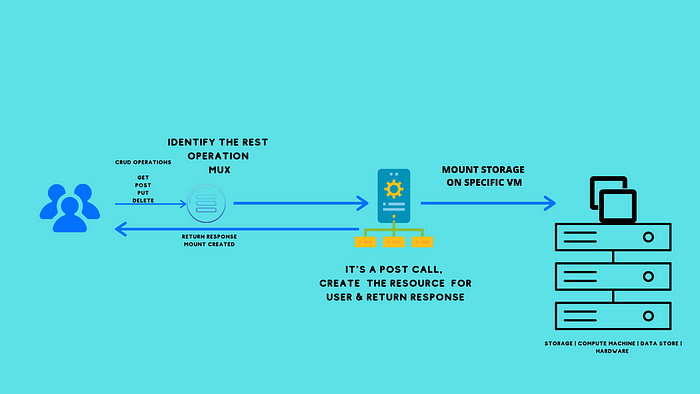
Fig 4 POST request example. User wants to create a resource which is identified by mux and then API server translates it to command and mount storage on specific VM.
Below is the code which is routing the user’s request :
Create a file called test_route_cloud_api.go as below:
Below code contains few major parts like :
- Import modules
- Main function where the execution will start
- Mux router will identify the type of request from user and redirect to specific subroutine. i.e CreateVM for POSt request CreateVM.
- http.Request and http.ResponseWriter respectively for sending request and receiving response.
Run the code using below command:
$go run test_route_cloud_api.go
And now you can test using POSTMAN tool or Browser.
If needed, install the missing modules using below command:
$go get github.com/gorilla/mux



In Fig 6. We are using remote command to launch a VM using command line virt-install command takes several argument like image location, networking parameters etc to launch a VM.
Conclusion: we can create golang services and handle user request and execute specific tasks according to the request type and send the response back to the user. In the next part i will be covering more into creating micro services and consuming APIs and get some real time results.